React hooks的几个demo
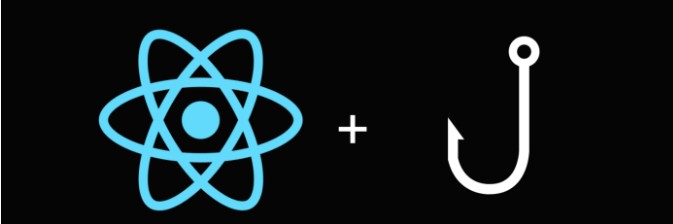
下面几个demo利于快速上手,反正我是万年计数器😂 😂
useState用法
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
import React, { useState } from 'react';
function Counter({ initialCount }) {
const [count, setCount] = useState(initialCount);
return (
<>
Count: {count}
<button onClick={() => setCount(initialCount)}>Reset</button>
<button onClick={() => setCount(prevCount => prevCount - 1)}>-</button>
<button onClick={() => setCount(prevCount => prevCount + 1)}>+</button>
</>
);
}
export default Counter
当initialCount需要计算时,可以写成一个函数,但只会在初始渲染时计算,比如下面initialCount=0的话,初始会变成1,但reset就会变成0
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
import React, { useState } from 'react';
function Counter({ initialCount }) {
const [count, setCount] = useState(()=>initialCount+1);
return (
<>
Count: {count}
<button onClick={() => setCount(initialCount)}>Reset</button>
<button onClick={() => setCount(prevCount => prevCount - 1)}>-</button>
<button onClick={() => setCount(prevCount => prevCount + 1)}>+</button>
</>
);
}
export default Counter
useEffect用法
- useEffect是在渲染DOM结束以后才会调用!
- useEffect不指定依赖的话每次渲染都会进行,
- useEffect指定空依赖的话挂载渲染一次,
- 尽量把有依赖的函数写在useEffect的作用域里
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
import React, { useState, useEffect } from 'react';
function Counter({ initialCount }) {
const [count, setCount] = useState(() => initialCount + 1);
useEffect(() => {//与 componentDidMount、componentDidUpdate
console.log("count发生变化了", count)
}, [count])
//用useEffect简单的一个防抖
useEffect(() => {
console.log("timer")
const timer = setTimeout(()=>{console.log("我发送了一个请求",count)}, 800)
return () => {
clearTimeout(timer)
console.log("clear")
}
}, [count])
return (
<>
Count: {count}
<button onClick={() => setCount(initialCount)}>Reset</button>
<button onClick={() => setCount(prevCount => prevCount - 1)}>-</button>
<button onClick={() => setCount(prevCount => prevCount + 1)}>+</button>
</>
);
}
export default Counter
useContext用法
偷个懒直接拿官网的,用法已经很清晰了
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
const themes = {
light: {
foreground: "#000000",
background: "#eeeeee"
},
dark: {
foreground: "#ffffff",
background: "#222222"
}
};
const ThemeContext = React.createContext(themes.light);
function App() {
return (
<ThemeContext.Provider value={themes.dark}>
<Toolbar />
</ThemeContext.Provider>
);
}
function Toolbar(props) {
return (
<div>
<ThemedButton />
</div>
);
}
function ThemedButton() {
const theme = useContext(ThemeContext);
return (
<button style={{ background: theme.background, color: theme.foreground }}>
I am styled by theme context!
</button>
);
}
export default App
useRef
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
import React, { useRef } from 'react';
function TextInputWithFocusButton() {
const inputEl = useRef(null);
const onButtonClick = () => {
// `current` 指向已挂载到 DOM 上的文本输入元素
inputEl.current.focus();
};
return (
<>
<input ref={inputEl} type="text" />
<button onClick={onButtonClick}>Focus the input</button>
</>
);
}
export default TextInputWithFocusButton
useImperativeHandle
useImperativeHandle 应当与 forwardRef 一起使用
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
import React, { useRef, forwardRef, useImperativeHandle, useState } from 'react';
function FancyInput(props, ref) {
const inputRef = useRef();
const [count, setCount] = useState(0)
useImperativeHandle(ref, () => ({
focus: () => {
inputRef.current.focus();
inputRef.current.value = count
}
}), [count]);
return (
<div>
<input ref={inputRef} />
<div>{count}</div>
<button onClick={() => setCount(prevCount => prevCount + 1)} >count</button>
</div>
)
}
const Son = forwardRef(FancyInput);
const Parent = props => {
const fancyInputRef = useRef();
return (
<div>
<Son ref={fancyInputRef} />
<button
onClick={() => fancyInputRef.current.focus()}
>父组件调用子组件的 focus</button>
</div>
)
}
export default Parent
useLayoutEffect
99%用不到 useLayoutEffect,useEffect都试试,useLayoutEffect可以消除闪动,碰到闪硕时可以尝试useLayoutEffect
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
import React, {
useState,
useLayoutEffect,useEffect
} from 'react';
const EffectRender = () => {
const [value, setValue] = useState(0);
useLayoutEffect(() => {
if (value === 0) {
setValue(10 + Math.random() * 200);
}
}, [value]);
console.log('render', value);
return (
< div onClick={() => setValue(0)} >
value: {value}
</div>
);
};
export default EffectRender
useDebugValue
仅开发工具中使用
useReducer,useCallback,useMemo之后做单独做介绍
(完)
0条看法
最新最后最热
等待你的评论