记面试碰到的一题
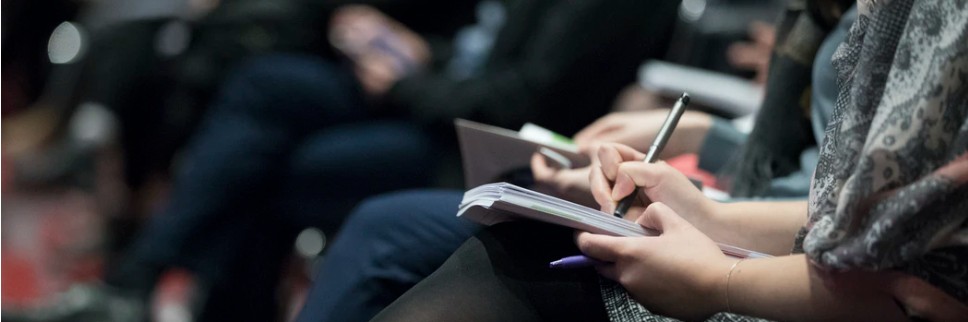
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
var array = [
{ id: 1, spec: 1 },
{ id: 5, spec: 2 },
{ id: 2, spec: 5 },
{ id: 2, spec: 10 },
{ id: 1, spec: 12 },
{ id: 3, spec: 12 },
{ id: 4, spec: 15 },
{ id: 3, spec: 16 }
]
转变为
[
{ id: 1, spec: [ 1, 12 ] },
{ id: 5, spec: [ 2 ] },
{ id: 2, spec: [ 5, 10 ] },
{ id: 3, spec: [ 12, 16 ] },
{ id: 4, spec: [ 15 ] }
]
面试时一开始的思路是把id迭代出来,再去重,然后再迭代原数组,判断id是否在id的数组里。然后filter那个id的元素,然后说着说着就有些乱了。。。用纸和笔写js的代码真是写不出来,功力不够啊
回到家,重新理一遍,用上vscode,撸出了下面的代码。
大概思路是出现过得id添加到了idarray里,也不用去重,然后迭代数组每一项,id出现过就创建一个新对象,没有就选出那个对象往数组后面添加东西,总的来看还是很复杂
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
function arrayChange(array){
let idArray = []
let newArray = []
for (let index = 0; index < array.length; index++) {
const element = array[index];
if (idArray.includes(element.id)) {
let curEle = newArray.filter(item => item.id === element.id)
curEle[0].spec.push(element.spec)
} else {
idArray.push(element.id)
let obj = {}
obj.id = element.id
obj.spec = []
obj.spec.push(element.spec)
newArray.push(obj)
}
}
return newArray
}
console.log(arrayChange(array))
下面是V友给出的解答
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
function arrayChange(array) {
return array.reduce((total, prev) => {
let item = total.find(item => item.id === prev.id)
if (!item) {
let ret = {
id: prev.id,
spec: [prev.spec]
}
total.push(ret)
} else {
item.spec.push(prev.spec)
}
return total
}, [])
}
此解法最佳
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
function solution(arr) {
const table =new Map();
for (const {id, spec} of arr) {
if (!table.has(id)) table.set(id, []);
table.get(id).push(spec);
}
return [...table].map(([id, spec]) => ({id, spec}));
}
console.log(solution(array))
很显然自己的Js基础比较差,借此学习一下Map的用法
- 1
- 2
- 3
- 4
let myMap=new Map()
myMap.set("a",1)
myMap.set("b",2)
console.log([...myMap]) //[ [ 'a', 1 ], [ 'b', 2 ] ]
MDN有比较详细的解释
https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Global_Objects/Map
(完)
0条看法
最新最后最热
等待你的评论